Battleships Server / Client for Education
I've been teaching a first year introductory module in Python programming for Engineering at Ulster University for a few years now. As part of the later labs I have let the students build a battleships game using Object Oriented Programming - with "Fleet" objects containing a list of "Ships" and so on where they could play on one computer against themselves. But I had often wanted to see if I could get as far as an on-line server application based on similar principles that the students could use. This would teach them client server fundamentals but also let them have fun playing against each other.
Last year I finally attained that goal. I built a very simple web-server using Python Django to manage players, games, and ships. I wanted to use Python partly because it's become my language of choice for web applications, but also because I wanted the code to be as accessible and understandable to the students as possible. I have initially placed very little emphasis on the User Interface of the server - this is partly deliberate because I wanted the students to have a strong incentive to develop this in the clients. I did however build a very simple admin view to let me see the games in progress. Additionally Django provides a very easy way to build admin views to manipulate objects. I have enabled this to let admins (well me) tweak data if I need to.
The Server
The server provides a very simple interface - an API - to let the students interact with the game. They can do this initially very directly using a web browser. They can simply type in specific web addresses to take certain actions. For instance
http://battleships.server.net/api/1.0/games/index/
will, for a battleships server installed on battleships.server.net (not a real server), list all the current active games. You'll note the api/1.0/ part of the URL. I did this so that in future years I could change the API and add new version numbers. That's partly the focus of a later section of this blog.
The output of all the API calls is actually encoded in JSON. This is still fairly human readable, and indeed some web browsers, like Firefox, will render this in a way that is easy to explore. Again, this makes it possible for the students to play the game with nothing more than a web browser and a certain amount of patience. This is good for exploration, but certainly not the easiest way to play. Here's some sample output from my real games server with my students (poor Brian).
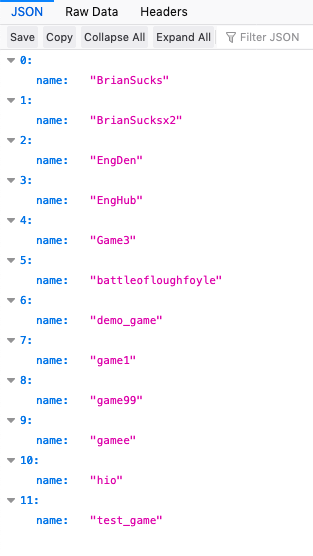
The code for the server and documentation about the API is all publicly available on my GitHub repository. You'll also find some basic installation instructions in case you'd like to run your own game server.
I did want to build some very basic views into the server, I built a more human version of the games list, and I also built this view which was designed only for admins - obviously in the hands of normal players it would make the game a bit pointless.
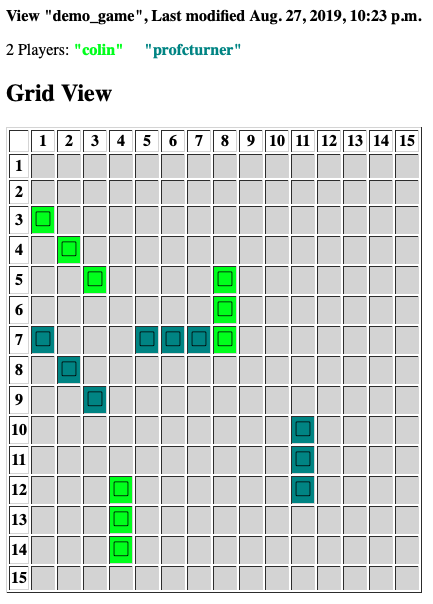
This allowed me to see a little of what was actually going on in the games my students were playing, as well as showing the current state of ships and who owns what, it also showed a bit more info - here for another game than that above:
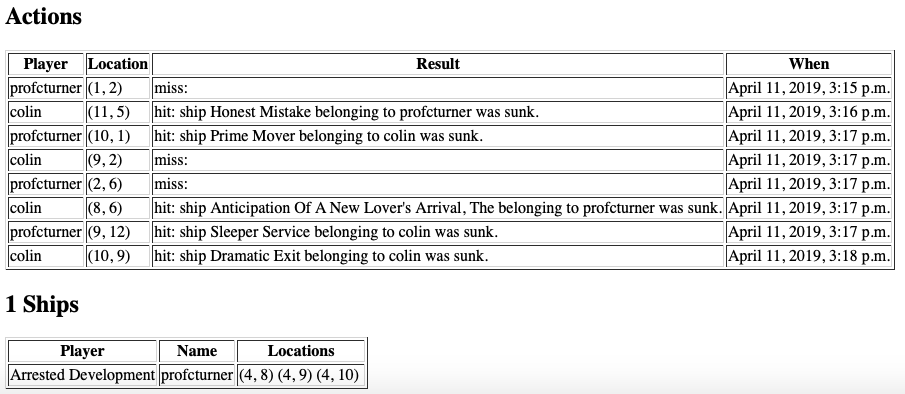
As you can see this shows some of the history of the game so far - this information is available to clients in the API, and likewise the list of surviving ships are shown. The API call for the ships still surviving only shows the ships for the player making the call, with a secret (password) that was generated when the player was created.
You may notice that the server automatically names ships with names taken from the Culture novels from Iain M. Banks.
The Client(s)
In this way the students are using their web browsers as a highly unsophisticated client to access the server. Actually playing the game this way will be frustrating - requiring careful URLs to be typed every time and noting the output for further commands. This is quite deliberate. It would be easy to build the web server to allow the whole game to be played seamlessly from a web browser - but this isn't the point - I want the students to experience building a client themselves and developing that.
For my module last year I gave the students a partially complete client, written in Python, using text only. Their aim for the lab was to complete the client. No client would be exactly the same, but they would all be written to work against the same server specification. In theory a better client will give a player an edge against others - a motivation for improvements. One student built the start of a graphical interface, some needed to be given more working pieces to complete their clients.
I've placed a more or less complete client on GitHub as well, but it could certainly do with a number of improvements. I may not make those since the idea of the project is to provide a focus for students to make these improvements.
What Went Well
The server worked reasonably well, and once I got going the code for automatic ship generation and placement worked quite nicely. I built a good number of unit tests for this which flushed out some problems, and which again are intended as a useful teaching resource.
I spent a reasonable amount of time building a server API that couldn't be too easily exploited. For instance, it's not possible for any player to be more than one move ahead of another to prevent brute force attacks by a client.
The server makes it relatively easy for as many players as one wants in a given game.
What Went Wrong
I made some missteps the first time around the block.
- the game grid's default size is too large - I made this configurable, but too large a grid makes the chances of quick hits much more remote - which the students need for motivation. I only really noticed this when I built my admin view shown above.
- in the initial game, any hit on a ship destroys it, there is no concept of health, and bigger ships are more vulnerable. This is arguably not a mistake, but is a deviation from expected behaviour.
What's Next
This year I may add another version of the API that adds health to ships so that multiple hits are needed for large ships. By moving all of this to a new version number in the URL as above, e.g.
http://battleships.server.net/api/2.0/games/index/
I hope to allow for improved versions of the game while still supporting (within reason) older clients accessing the original version.
I might also add admin calls to tweak the number of ships per player and size of the ships. There are defaults in the code, but these can easily be overriden.
Shall We Play A Game?
There's absolutely no reason the clients need to be written in Python. There's no reason not to write one in C++ or Java, or to build a client that runs on Android or iOS phones. There's no reason that first year classes with a text mode Python client can't play against final year students using an Android client.
If you are teaching in any of these languages and feel like writing new clients against the server please do. If you feel like making your clients open source to help more students (and lecturers) learn more, that would be super too.
If you have some improvements in mind for the server, do feel free to fork it, or put in pull requests.
If you or your class do have a go at this, I'd love to hear from you.